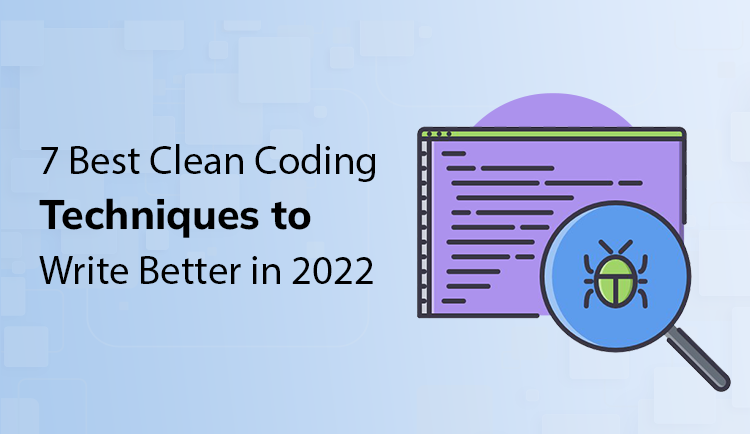
Clean Coding Techniques: 7 Best Ways to Write Better in 2022
Software engineering is more than just learning a programming language and creating software. Software engineers are expected to produce high-quality or good software, which is only possible with clean coding techniques.
Those who do not adhere to clean coding practices do fail to create good software.
More often than, beginners have questions such as:
- What constitutes clean coding?
- Why do software developers fail to produce clean code?
- How to write more reliable and clean code?
So, if you also have the same questions in mind, read this article.
In this article, we’ll answer all your questions one by one. So, stay tuned!
What constitutes clean coding?
If the code is simple to understand and modify, it is almost certain that the program is good, and engineers will enjoy working on the project. However, here are the main features of clean code:
It should be simple and easy to comprehend:
This goes without saying. When we talk about clean code principles, the simplicity of code tops the list. Your code should adhere to the notion of single accountability (SRP).
It should be readable:
When writing a code, keep in mind that your code should be as easy to read as poetry.
It should be neat:
It is one of the most important clean coding practices. Your code should be neat and enjoyable to be called a clean code.
It should be easy to comprehend, modify, and manage:
You may need to alter your code a number of times. Hence, it is crucial to create code that can be modified easily. Unnecessary notations make a code complicated, which makes managing them a task.
It should pass all tests:
Clean code is full of precise abstract ideas and simple control structures that pass all tests in one go.
Let’s now move to our next point of contention,
Why do software developers fail to produce clean code?
Beginners often fail to see or comprehend a level of intricacy to software development. Numerous clean coding practices in the clean code architecture are difficult for new developers and non-developers to comprehend.
Most developers, especially novices, have no knowledge of what they are doing or why they are doing it. This is referred to as the Dunning-Kruger effect, which leads to poor software development.
Another common reason is the pressure of meeting deadlines, due to which developers refrain from developing clean code. They rush to move quicker, but what actually happens is that they end up going slower as a result.
It leads to the creation of additional bugs, which they must later repair by reverting to the original piece of code. This approach consumes significantly more time than the time spent writing the code itself.
Know that clean coding is not just a need; it’s a necessity today. Whether you’re a beginner or an expert programmer, you should always aim to write clean code. The quality of your code totally depends on how much you adhere to clean code principles.
Thus, build a program that other developers can understand and not criticize you every time they try to debug your terrible code.
It’s now time to see how clean coding is accomplished…
How to Write More Reliable and Clean Code?
1. Stick to Basics
First and foremost, keep your code as simple and comprehensible as possible. Frequently, software developers overcomplicate things, which simply hamper the process of clean coding.
By adhering to clean code practices, you may keep things simple, produce higher-quality code, solve problems more quickly, work more effectively with other developers, and maintain a more extensible and explicit code base.
2. Make Use of Meaningful Naming
You’ll be writing a lot of variables, functions, classes, arguments, modules, packages, and directory names. Make it a practice to name your variables meaningfully in your code.
Whatever names you use in your code, it should serve three purposes:
- Describe what the code does
- Why it exists; and
- How it is utilized
For instance: int b; // User count.
In the above example, you must provide a comment alongside the variable’s name declaration, which is not a trait of good programming. The name you give your code should be self-explanatory. It should contain information on the purpose of a variable, function, or method.
Thus, a more appropriate variable name would be int user_count in the preceding example.
While choosing meaningful names takes time, it results in much cleaner and easier-to-read code for both other developers and yourself. Additionally, attempt to keep the names to three or four words.
3. Single Responsibility Principle
More often than not, beginners write a function that can handle and accomplish multiple tasks. It confuses developers and presents issues when they need to patch errors or locate a piece of code.
You should always follow the Single Responsibility Principle for writing clean coding. This principle argues that each class should have only one responsibility and one goal. This indicates a class has just one job; hence, it should have only one reason to change.
Our goal is to avoid unrelated items that are harder to maintain. We should break up the class into smaller classes, each dealing with a distinct issue. Surely an error will be easy to spot.
Here is an example:
Consider a class that has code that modifies text. Its job will only be to manipulate text.
public class TextManipulator {
private String text;
public TextManipulator(String text) {
this.text = text;
}
public String getText() {
return text;
}
public void appendText(String newText) {
text = text.concat(newText);
}
public String findWordAndReplace(String word, String replacementWord) {
if (text.contains(word)) {
text = text.replace(word, replacementWord);
}
return text;
}
public String findWordAndDelete(String word) {
if (text.contains(word)) {
text = text.replace(word, "");
}
return text;
}
public void printText() {
System.out.println(textManipulator.getText());
}
}
Thus, when developing a function, keep the following two points in mind to ensure that it is clean and simple to understand…
- They should be small in size.
- They should focus exclusively on one task and perform it effectively.
- They should be cohesive.
- They should not have more than three arguments.
Adhering to these clean code practices, you may significantly improve the readability of your code and enable other developers to comprehend or build a new feature.
4. Focus on Code Formatting
A code that is difficult to comprehend is considered a bad code. As a developer, whether beginner or expert, your aim should be to focus on creating readable code. Code formatting comes to your rescue when it comes to writing clean code.
Code formatting is one of the most important clean code principles since it enables any markup to be more easily understood, maintained, and troubleshoot. It offers developers numerous options to communicate their intent effectively to a reader.
Many newcomers make the error of writing their code in a single line without using adequate whitespace, indentation, or line breaks, which is one of the poorest clean coding practices. This results in a disorganized and difficult-to-maintain codebase.
Also, it wastes the time of other developers who have access to your code and the authority to work with it. Hence, it is imperative to pay close attention to the formatting of your code for clean coding. Not only will it save time and effort, but it also will make managing it a breeze for all.
Therefore, ensure that your code is appropriately indented, spaced, and has line breaks to make it readable to others.
Here are the examples of both Bad code and Good Code
Bad Code
class CarouselRightArrow extends Component{
render(){
return (
<a
href="#"
className="carousel__arrow carousel__arrow--left"
onClick={this.props.onClick}
>
<span className="fa fa-2x fa-angle-left"/>
</a> );
}
};
Good Code
class CarouselRightArrow extends Component {
render() {
return (
<a
href="#"
className="carousel__arrow carousel__arrow--left"
onClick={this.props.onClick}
>
<span className="fa fa-2x fa-angle-left" />
</a>
);
}
};
Code formatting is an art that can help software developers become masters of their field!
5. Incorporate Comments into Your Code
This is one of the most imperative clean coding standards; however, most coders overlook it. If you want to perfect clean coding, make incorporating comments into your code a ritual.
It makes updating, debugging analysis, and other post-programming procedures easier and faster. Furthermore, if you’re working in a team, providing comments in the code makes it easy for your peers to understand your code vision.
BUT, BUT, BUT DON’T OVERDO IT!
Developers, especially beginners, tend to overdo it. While comments are handy for explaining what the code does; however, they also need extra upkeep of your code.
Often when a code is moved about during development, the comment remains in the same location, creating confusion among other developers. As a result, it is a suggestion to avoid making superfluous remarks, make only wherever it is necessary.
For example, if you’re working with other team members and need to explain specific behavior, you can use comments to explain your code; nevertheless, avoid using comments where they are redundant.
6. Write Unit Tests
Unit testing is critical for good software development. Unit tests ensure that your code remains flexible, maintainable, and reusable. Without testing, every modification has the potential to introduce an issue.
Regardless of how adaptable your clean coding architecture is or how well partitioned your design is, without testing, you will be hesitant to make changes for fear of introducing undetected defects.
However, unit testing just eliminates all such fears. The greater your test coverage, the less fearful you will be.
In software development, there is a process called Test Driven Development or TDD or Red-Green-Refactor that converts requirements into particular test cases and subsequently improves the product to pass additional tests.
Test Driven Development’s Three Principles
Write a failing test:
You are not permitted to create production code until you have written a unit test that fails.
Make the test pass:
You may not write more unit tests than are necessary to fail, and failing to compile is considered failing.
Refactor as necessary:
You are not permitted to create any additional production code beyond what is required to pass the single failed unit test.
7. Organize your Project
Last but not least, organize your project as you work on it. Clean coding is, without a doubt, an arduous undertaking. When software is being developed, a large number of files and directories are produced and removed, making it difficult for other developers to understand and work on the project effectively.
As a result, it is recommended that you build a consistent file structure from the beginning. A well-organized folder will help you, and your fellow developers understand and manage the project without wasting any time.
Final Words
So, this is all about Clean Coding techniques to write better in 2022. Hopefully, this article has been enlightening for you and will help you write clean code.
Remember that writing clean code is an art that you develop with regular practice. Aside from following the 7 best clean coding practices outlined above, having patience is essential for developing GOOD SOFTWARE. So, be patient and adhere to clean coding practices to master the art!