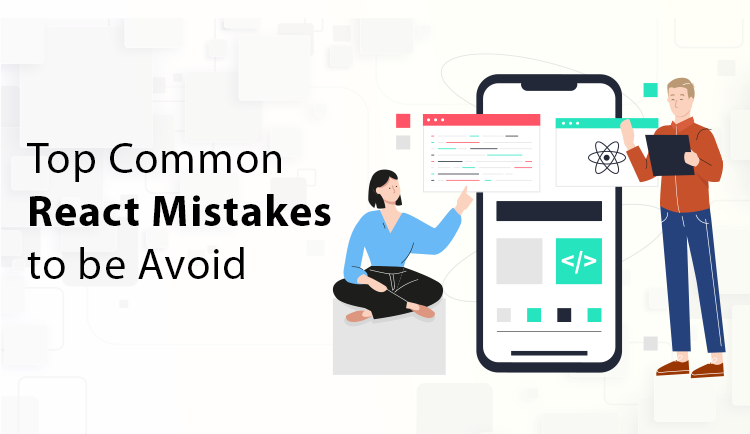
Top 5 Common React Mistakes To Be Avoided At All Costs
To err is human; however, that’s no excuse when using React to craft a one-of-a-kind user experience. Mistakes are pretty common among developers. It’s possible to brush off certain react mistakes; however, others can wreak havoc while mobile app development with react.
The first step for aspiring developers willing to create distinctive designs is to learn react common mistakes and how to avoid them. And in this post, we shall discuss some of the common react mistakes that you MUST AVOID in the process.
Let’s get started…
5 Most Common React Mistakes Developers MUST AVOID
1. Not Creating Enough Components
Constructing a complete page using a single component that contains all the UI elements is the most common react mistake.
When working with React, it’s typical for developers to make the error of not making enough of the key components.
Undoubtedly, with react, you can build huge components that do multiple actions. However, it is recommended that you keep components short, with each performing a single purpose. Not only will it save time, but it will also help you in debugging because you can pinpoint exactly which parts are causing problems.
Here is an example:
//./components/TodoList.js
import React from 'react';
import { useTodoList } from '../hooks/useTodoList';
import { useQuery } from '../hooks/useQuery';
import TodoItem from './TodoItem';
import NewTodo from './NewTodo';
const TodoList = () => {
const { getQuery, setQuery } = useQuery();
const todos = useTodoList();
return (
<div>
<ul>
{todos.map(({ id, title, completed }) => (
<TodoItem key={id} id={id} title={title} completed={completed} />
))}
<NewTodo />
</ul>
<div>
Highlight Query for incomplete items:
<input value={getQuery()} onChange={e => setQuery(e.target.value)} />
</div>
</div>
);
};
export default TodoList;
2. Modifying the State Directly
This is one of the most common react beginner mistakes who are just starting out with React. It can wreak havoc on your software, causing (possibly) difficult-to-reproduce errors and (unwanted) side effects.
You shouldn’t directly edit state in React; doing so can lead to bugs and performance concerns that can be difficult to debug if they occur.
Here is an example of a pf state mutation that you should avoid at all costs:
export default function App() {
const [todos, setTodos] = React.useState([]);
return (
<div>
<h1>Todo List</h1>
<TodoList todos={todos} />
<AddTodo setTodos={setTodos} todos={todos} />
</div>
);
}
function AddTodo({ setTodos, todos }) {
function handleAddTodo(event) {
event.preventDefault();
const text = event.target.elements.addTodo.value;
const todo = {
id: 4,
text,
done: false
};
const newTodos = todos.concat(todo);
setTodos(newTodos);
}
return (
<form onSubmit={handleAddTodo}>
<input name="addTodo" placeholder="Add todo" />
<button type="submit">Submit</button>
</form>
);
}
After adding the new task, we are doing what has to be done and adding it to the todos array. Todos in the TodoList widget will be refreshed as a result.
The todos array is not required to be passed down from the parent state to the child state because the child state is based on the parent state.
Instead, we can write a function inside setState to retrieve the previous todos state. This function’s return value will become the new default.
This means that the setTodos function is all that has to be inherited for a state to be updated correctly.
export default function App() {
const [todos, setTodos] = React.useState([]);
return (
<div>
<h1>Todo List</h1>
<TodoList todos={todos} />
<AddTodo setTodos={setTodos} />
</div>
);
}
function AddTodo({ setTodos }) {
function handleAddTodo(event) {
event.preventDefault();
const text = event.target.elements.addTodo.value;
const todo = {
id: 4,
text,
done: false
};
setTodos(prevTodos => prevTodos.concat(todo));
}
return (
<form onSubmit={handleAddTodo}>
<input name="addTodo" placeholder="Add todo" />
<button type="submit">Submit</button>
</form>
);
}
3. Accessing React State Immediately After Setting It
Accessing a setState() immediately after it has been set is yet another react common mistake.
setState() is the function to utilize when you need to make changes to the state of your application. And it’s a no-brainer that setState is asynchronous.
This means that the changes won’t show up until the next render, at the earliest.
Similarly, this is the case with useState() (React Hooks) as well.
React can combine together several update calls to improve efficiency further.
The entire process is automated. Therefore, retrieving a state value immediately after setting it may not always produce the best results.
Here is an example:
class App extends Component {
state = { clickCounts: {} };
onClick = e => {
e.persist();
const clickCounts = { ...this.state.clickCounts };
let clickCount = +clickCounts[e.target.name] || 0;
clickCounts[e.target.name] = ++clickCount;
this.setState({ clickCounts };
console.log(
`Button Name (outside) = ${e.target.name}`,
this.state.clickCounts
);
};
render() {
const buttons = [1, 2, 3].map(id => (
<button
key={id}
name={`button${id}`}
onClick={this.onClick}
>{`Button #${id}`}</button>
));
return <div className="App">{buttons}</div>;
}
}
As setState is an action, you can just wait until React sets the value. setTimeout can be used to delay access to the updated state for a period of time.
setState(updater[, callback])
Here is the updated code:
onClick = e => {
e.persist();
const clickCounts = { ...this.state.clickCounts };
let clickCount = +clickCounts[e.target.name] || 0;
clickCounts[e.target.name] = ++clickCount;
// - From this,
// this.setState({ clickCounts });
// + To this
this.setState({ clickCounts }, () =>
console.log(
`Button Name (inside callback) = `,
this.state.clickCounts
)
);
console.log(
`Button Name (outside callback) = ${e.target.name}`,
this.state.clickCounts
);
};
4. Not Using Key on a Listing Element
Not using the key on a listing component is one of the most prevalent react mistakes developers commit.
A key is needed to provide each array element with a distinct identity. It tells React what has been modified (added, removed, rearranged).
Not using a key can help in some scenarios. However, when working with huge lists, you may need help with making changes or removing items.
React tracks each DOM element in a list. It is necessary in order to determine what has changed in the corresponding list item.
There are scenarios in which we must render a list of things.
Here is an example of one such code:
const lists = ['one', 'two', 'three'];
render() {
return (
<ul>
{lists.map(listNo =>
<li>{listNo}</li>)}
</ul>
);
}
Adding a key to each element of your list is essential.
Here is how it looks:
<ul>
{lists.map(listNo =>
<li key={listNo}>{listNo}</li>)}
</ul>
5. Manage All of Your App’s States with Redux or Flux
Last but not least, using Redux or Flux to manage your App’s states is again a react mistake.
More often than not, React app developers utilize Redux or Flux for global state management in their more complex projects. Undoubtedly, it helps as it allows different areas of the program to share the same state.
However, it is not recommended that you utilize Redux or Flux to handle every state in your program.
When it comes to common patterns, especially those involving asynchronous data fetching, both Redux and Flux require a lot of boilerplate code.
While libraries like Alt and Fluxxor’s implementation of Flux provide nearly all of the functionality that Redux requires, it places emphasis on flexibility over convenience.
Because Redux makes assumptions about your state, such assumptions may be unintentionally disregarded, which could be a drawback for some developers.
Redux or Flux can be used in the following scenarios:
- If there are a lot of component-to-component interactions that you don’t want to transmit to the server,
- If multiple parts need access to the same information but you don’t want to repeatedly retrieve it.
- If your App has some API data that will change many parts based on how users interact with it.
The Final Verdict
So, there you have it: the top 5 react mistakes that every app developer, budding or seasoned, must avoid. Avoiding these react common mistakes is the first step toward clean coding and developing a better-performing application.
If you want to enhance your react skills or are looking for the best react development company, then Annexlogics is your best bet!
Our team of expert developers can help you create the app of your dream in no time!
Contact us for more details!
Thanks for reading! Hopefully, this piece has been enlightening for you and will help you avoid react mistakes.
Stay tuned for such insightful posts!